Guide to Laravel Actions
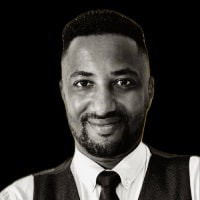
- Published on
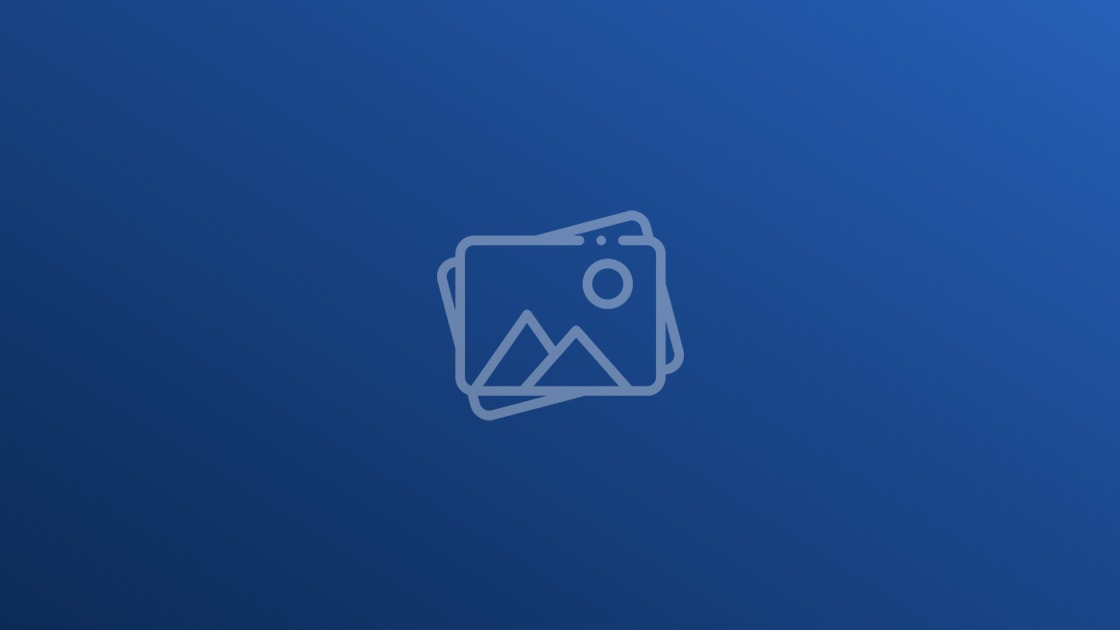
Laravel Actions is a design pattern and an emerging approach in Laravel development that helps you organize your code into single, reusable classes, promoting clarity and maintainability.
What are Laravel Actions?
Laravel Actions encapsulate a single operation, such as creating a user, sending an email, or processing an order, into its own dedicated class. This keeps your controllers and service classes clean and focused.
Why Use Actions in Laravel?
- Single Responsibility Principle (SRP): Each action class handles one specific task.
- Reusability: Actions can be reused across the application.
- Testability: Each action can be tested independently.
- Readability: Code is easier to read and maintain.
Creating an Action Class
Here is an example of how to create an action class manually:
namespace App\Actions;
use App\Models\User;
class CreateUserAction
{
public function execute(array $data): User
{
return User::create($data);
}
}
Invoking an Action
Actions can be called easily from controllers or other parts of the application:
use App\Actions\CreateUserAction;
$user = (new CreateUserAction())->execute($request->all());
Using Laravel Packages for Actions
Some popular Laravel packages for managing actions include:
- Laravel Actions by Loris Leiva – Adds more structure and features to your actions.
- Laravel Zero Actions – Focused on console applications.
Best Practices
- Use descriptive names for your action classes.
- Keep each action focused on a single task.
- Inject dependencies through the constructor.
- Ensure your actions are stateless.
Conclusion
Laravel Actions can significantly improve the structure of your application, making your code more modular, reusable, and testable. Consider incorporating actions into your Laravel projects for better code organization and maintainability.
Happy Coding with Laravel! 🚀